1、程序簡介
該程序是基于OpenHarmony的C++公共基礎類庫的安全關聯容器:SafeMap。
OpenHarmony提供了一個線程安全的map實現。SafeMap在STL map基礎上封裝互斥鎖,以確保對map的操作安全。
本案例主要完成如下工作:
創建1個子線程,負責每秒調用EnsureInsert()插入元素;
創建1個子線程,負責每秒調用Insert()插入元素;
創建1個子線程,負責每秒調用Erase()刪除元素;
創建1個子線程,負責每秒調用FindOldAndSetNew()替換元素的值;
主線程等待上述線程結束,Iterate()和Find()查看所有元素;
主線程等待上述線程結束,清空SafeMap,并調用IsEmpty()查看是否確實是空。
2、基礎知識
C++公共基礎類庫為標準系統提供了一些常用的C++開發工具類,包括:
文件、路徑、字符串相關操作的能力增強接口
安全數據容器、數據序列化等接口
各子系統的錯誤碼相關定義
2.1、添加C++公共基礎類庫依賴
修改需調用模塊的BUILD.gn,在external_deps或deps中添加如下:
ohos_shared_library("xxxxx") { ... external_deps = [ ... # 動態庫依賴(可選) "c_utils:utils", # 靜態庫依賴(可選) "c_utils:utilsbase", # Rust動態庫依賴(可選) "c_utils:utils_rust", ] ...}
一般而言,我們只需要填寫"c_utils:utils"即可。
2.2、SafeMap頭文件
C++公共基礎類庫的safemap頭文件在://commonlibrary/c_utils/base/include/safe_map.h
可在源代碼中添加如下:
#include
2.3、OHOS::SafeMap接口說明
2.3.1、SafeMap
構造函數。
SafeMap();SafeMap(const SafeMap& rhs);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
rhs | SafeMap | 復制SafeMap的類對象 |
2.3.2、~SafeMap
析構函數。
~SafeMap();
2.3.3、Clear
刪除map中存儲的所有鍵值對。
void Clear();
2.3.4、EnsureInsert
在map中插入元素。
void EnsureInsert(const K& key, const V& value);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
key | K | 需要插入元素的關鍵字 |
value | V | 需要插入元素的值 |
2.3.5、Erase
刪除map中鍵為key的鍵值對。
void Erase(const K& key);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
key | K | 需要刪除元素的關鍵字 |
2.3.6、Find
在map中查找元素。
bool Find(const K& key, V& value);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
key | K | 需要查找元素的關鍵字 |
value | V | 需要查找元素的值 |
返回值說明:
類型 | 返回值說明 |
---|---|
bool | true表示成功,false表示失敗 |
2.3.7、FindOldAndSetNew
在map中查找元素并將key對應的oldValue替換為newValue。
boolFindOldAndSetNew(constK&key,V&oldValue,constV&newValue);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
key | K | 需要替換元素的關鍵字 |
oldValue | V | 需要替換元素的原始值 |
newValue | V | 需要替換元素的新值 |
返回值說明:
類型 | 返回值說明 |
---|---|
bool | true表示成功,false表示失敗 |
2.3.8、Insert
在map中插入新元素。
bool Insert(const K& key, const V& value);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
key | K | 需要插入元素的關鍵字 |
value | V | 需要插入元素的原始值 |
返回值說明:
類型 | 返回值說明 |
---|---|
bool | true表示成功,false表示失敗 |
2.3.9、IsEmpty
判斷map是否為空。
bool IsEmpty();
返回值說明:
類型 | 返回值說明 |
---|---|
bool | true表示空,false表示非空 |
2.3.10、Iterate
遍歷map中的元素。
bool Iterate(const SafeMapCallBack& callback);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
callback | SafeMapCallBack | 遍歷執行函數 |
2.3.11、operator=
SafeMap賦值。
SafeMap& operator=(const SafeMap& rhs);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
rhs | SafeMap& | 被賦值的SafeMap類對象 |
返回值說明:
類型 | 返回值說明 |
---|---|
SafeMap | 賦值的SafeMap類對象 |
2.3.12、operator[]
SafeMap索引。
V& operator[](const K& key);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
key | K& | 元素的關鍵字 |
返回值說明:
類型 | 返回值說明 |
---|---|
V& | 返回元素的值 |
2.3.13、Size
獲取map的size大小。
int Size();
返回值說明:
類型 | 返回值說明 |
---|---|
int | map的size大小 |
3、程序解析
3.1、創建編譯引導
在上一級目錄BUILD.gn文件添加一行編譯引導語句。
import("http://build/ohos.gni")
group("samples") { deps = [ "a26_utils_safemap:utils_safemap", # 添加該行 ]}
"a26_utils_safemap:utils_safemap",該行語句表示引入 參與編譯。
3.2、創建編譯項目
創建a26_utils_safemap目錄,并添加如下文件:
a26_utils_safemap├── utils_safemap_sample.cpp # .cpp源代碼├── BUILD.gn # GN文件
3.3、創建BUILD.gn
編輯BUILD.gn文件。
import("http://build/ohos.gni")ohos_executable("utils_safemap") { sources = [ "utils_safemap_sample.cpp" ] include_dirs = [ "http://commonlibrary/c_utils/base/include", "http://commonlibrary/c_utils/base:utils", "http://third_party/googletest:gtest_main", "http://third_party/googletest/googletest/include" ] external_deps = [ "c_utils:utils" ] part_name = "product_rk3568" install_enable = true}
注意:
(1)BUILD.gn中所有的TAB鍵必須轉化為空格,否則會報錯。如果自己不知道如何規范化,可以:
# 安裝gn工具sudo apt-get install ninja-buildsudo apt install generate-ninja# 規范化BUILD.gngn format BUILD.gn
3.4、創建源代碼
3.4.1、創建SafeMap
#include // SafeMap的頭文件
// 定義SafeMap變量static OHOS::SafeMap
3.4.2、創建線程池并設置
int main(int argc, char **argv){ OHOS::ThreadPool threads("name_rwlock_threads"); string str_name; ...... threads.SetMaxTaskNum(128); threads.Start(4); ......}
3.4.3、啟動4個子線程,并等待結束
調用AddTask()添加子線程,并調用Stop()等待所有子進程結束。
// 開啟子線程,使用EnsureInsert插入元素str_name = "Thread_EnsureInsert";auto task_ensure_insert = std::bind(map_ensure_insert, str_name);threads.AddTask(task_ensure_insert);
// 開啟子線程,使用Insert插入元素str_name = "Thread_Insert";auto task_insert = std::bind(map_insert, str_name);threads.AddTask(task_insert);
// 開啟子線程,使用erase刪除元素str_name = "Thread_Erase";auto task_erase = std::bind(map_erase, str_name);threads.AddTask(task_erase);
// 開啟子線程,使用FindOldAndSetNew替換元素的值str_name = "Thread_FindOldAndSetNew";auto task_findold_and_setnew = std::bind(map_findold_and_setnew, str_name);threads.AddTask(task_findold_and_setnew);
// 設置結束,并等待結束threads.Stop();cout << "Threads Stop" << endl;
3.4.4、編寫SafeMap.EnsureInsert()插入元素
void map_ensure_insert(const string& name){ int key = 0; string value = ""; for (int i = 0; i < (sizeof(m_map1_insert) / sizeof(struct MapInfo)); i++) { key = m_map1_insert[i].key; value = m_map1_insert[i].str; m_safemap.EnsureInsert(key, value); cout << name << ": insert successful and key = " << key << " and value = " << value << endl; sleep(1); }}
3.4.5、編寫SafeMap.Insert()插入元素
void map_insert(const string& name){ int key = 0; string value = ""; for (int i = 0; i < (sizeof(m_map2_insert) / sizeof(struct MapInfo)); i++) { key = m_map2_insert[i].key; value = m_map2_insert[i].str; if (m_safemap.Insert(key, value) == false) { cout << name << ": insert failed and key = " << to_string(key) << " and value = " << value << endl; } else { cout << name << ": insert successful and key = " << to_string(key) << " and value = " << value << endl; } sleep(1); }}
3.4.6、編寫SafeMap.Erase()刪除元素
void map_erase(const string& name){ int key = 0; string value = ""; for (int i = 0; i < (sizeof(m_map2_insert) / sizeof(struct MapInfo)); i++) { key = m_map2_insert[i].key; m_safemap.Erase(key); cout << name << ": Erase successful and key = " << to_string(key) << endl; sleep(1); }}
3.4.7、編寫SafeMap.FindOldAndSetNew()替換元素的值
void map_findold_and_setnew(const string& name){ int key = 0; string old_value = ""; string new_value = ""; for (int i = 0; i < (sizeof(m_map1_insert) / sizeof(struct MapInfo)); i++) { key = m_map1_reset[i].key; old_value = ""; new_value = m_map1_reset[i].str; if (m_safemap.FindOldAndSetNew(key, old_value, new_value) == false) { cout << name << ": FindOldAndSetNew failed and key = " << to_string(key) << " and old_value = " << old_value << endl; } else { cout << name << ": FindOldAndSetNew successful and key = " << to_string(key) << " and old_value = " << old_value << " and new_value = " << new_value << endl; } sleep(1); }}
3.4.8、編寫枚舉所有元素
主要分為如下兩種方法:
(1)調用SafeMap.Iterate()
void map_iterate_print(const int key, string& value){ cout << "key = " << to_string(key) << ", value = " << value << endl;}
int main(int argc, char *argv[]){ ...... cout << "SafeMap Iterate: " << endl; m_safemap.Iterate(map_iterate_print); ......}
(2)調用SafeMap.Find()
void map_find_print(){ int key = 0; string value = ""; for (int i = 0; i < (sizeof(m_map1_insert) / sizeof(struct MapInfo)); i++) { key = m_map1_insert[i].key; value = ""; if (m_safemap.Find(key, value)) { cout << "key = " << to_string(key) << ", value = " << value << endl; } } for (int i = 0; i < (sizeof(m_map2_insert) / sizeof(struct MapInfo)); i++) { key = m_map2_insert[i].key; value = ""; if (m_safemap.Find(key, value)) { cout << "key = " << to_string(key) << ", value = " << value << endl; } }}
3.4.9、清空SafeMap
int main(int argc, char *argv[]){ ...... cout << "SafeMap Clear" << endl; m_safemap.Clear(); cout << "SafeMap IsEmpty: " << m_safemap.IsEmpty() << endl; ......}
4、編譯步驟
進入OpenHarmony編譯環境,運行命令:
hb build -f
5、運行結果
# utils_safemapThread_EnsureInsert: insert successful and key = 1 and value = aaaThread_Erase: Erase successful and key = Thread_FindOldAndSetNew: FindOldAndSetNew successful and key = 1 and old_value = aaa and new_value = abc101Thread_Insert: insert successful and key = 101 and value = 111Thread_EnsureInsert: insert successful and key = Thread_FindOldAndSetNew: FindOldAndSetNew successful and key = 2 and old_value = bbb and new_value = bcdThread_Insert: insert successful and key = 102 and value = 2222 and value = bbb
Thread_Erase: Erase successful and key = 102Thread_EnsureInsert: insert successful and key = 3 and value = cccThread_FindOldAndSetNew: FindOldAndSetNew successful and key = 3 and old_value = ccc and new_value = cdeThread_Insert: insert successful and key = 103 and value = 333Thread_Erase: Erase successful and key = 103Thread_EnsureInsert: insert successful and key = 4 and value = dddThread_Insert: insert successful and key = 104Thread_FindOldAndSetNew and value = : FindOldAndSetNew successful and key = 4444 and old_value = ddd and new_value = def
Thread_Erase: Erase successful and key = 104Thread_EnsureInsert: insert successful and key = 5 and value = eeeThread_Insert: insert successful and key = 105 and value = 555Thread_FindOldAndSetNew: FindOldAndSetNew successful and key = 5 and old_value = eee and new_value = efgThread_Erase: Erase successful and key = 105Thread_EnsureInsert: insert successful and key = 6 and value = fffThread_FindOldAndSetNew: FindOldAndSetNew successful and key = 6 and old_value = fff and new_value = fghThread_Insert: insert successful and key = 106 and value = 666Thread_Erase: Erase successful and key = 106Thread_EnsureInsert: insert successful and key = 7 and value = gggThread_FindOldAndSetNew: FindOldAndSetNew successful and key = 7 and old_value = ggg and new_value = ghiThread_Erase: Erase successful and key = 107Thread_Insert: insert successful and key = 107 and value = 777Thread_EnsureInsert: insert successful and key = 8 and value = hhhThread_FindOldAndSetNew: FindOldAndSetNew successful and key = 8 and old_value = hhh and new_value = hijThread_Erase: Erase successful and key = 108Thread_Insert: insert successful and key = 108 and value = 888Thread_EnsureInsert: insert successful and key = 9 and value = iiiThread_FindOldAndSetNew: FindOldAndSetNew successful and key = 9 and old_value = iii and new_value = ijkThread_Erase: Erase successful and key = 109Thread_Insert: insert successful and key = 109 and value = 999Thread_EnsureInsert: insert successful and key = 10 and value = jjjThread_FindOldAndSetNew: FindOldAndSetNew successful and key = 10 and old_value = jjj and new_value = jklThread_Erase: Erase successful and key = 110Thread_Insert: insert successful and key = 110 and value = 000Threads StopSafeMap Iterate:key = 1, value = abckey = 2, value = bcdkey = 3, value = cdekey = 4, value = defkey = 5, value = efgkey = 6, value = fghkey = 7, value = ghikey = 8, value = hijkey = 9, value = ijkkey = 10, value = jklkey = 108, value = 888key = 109, value = 999key = 110, value = 000SafeMap Find:key = 1, value = abckey = 2, value = bcdkey = 3, value = cdekey = 4, value = defkey = 5, value = efgkey = 6, value = fghkey = 7, value = ghikey = 8, value = hijkey = 9, value = ijkkey = 10, value = jklkey = 108, value = 888key = 109, value = 999key = 110, value = 000SafeMap ClearSafeMap IsEmpty: 1#
-
MAP
+關注
關注
0文章
49瀏覽量
15235 -
Safe
+關注
關注
0文章
6瀏覽量
7276 -
OpenHarmony
+關注
關注
25文章
3771瀏覽量
17062
發布評論請先 登錄
相關推薦
基于OpenHarmony標準系統的C++公共基礎類庫案例:ThreadPoll
基于OpenHarmony標準系統的C++公共基礎類庫案例:Semaphore
基于OpenHarmony標準系統的C++公共基礎類庫案例:rwlock
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeMap
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeQueue
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeStack
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeBlockQueue
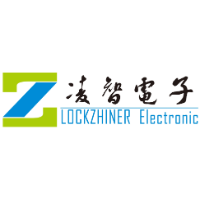
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeStack
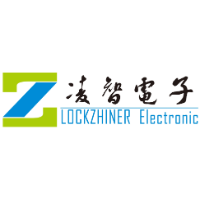
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeQueue
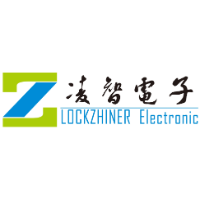
基于OpenHarmony標準系統的C++公共基礎類庫案例:rwlock
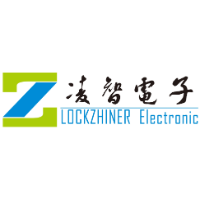
評論