函數原型
C++中std::tie函數的作用就是從元素引用中生成一個tuple元組,其在頭文件中定義,其函數原型如下:
template< class... Types >
std::tuple< Types&... > tie( Types&... args ) noexcept; //C++11起, C++14前
template< class... Types >
constexpr std::tuple< Types&... > tie( Types&... args ) noexcept;//C++14起
其中參數 args 為構造 tuple 所用的零或更多左值參數。其返回值為含的std::tuple對象。
作用和用法
1. 解包 tuple 和 pair
std::tie 可以用于解包 tuple 和 pair,因為 std::tuple 擁有從 pair 的轉換賦值。
注:元組tuple可以將不同類型的元素存放在一起,可以理解為pair的擴展(pair只能包含兩個元素,而tuple可以多個)。
std::tuple擁有從 pair 的轉換賦值的主要原因就是:tuple的實現中重載了 operator=,其部分原型如下:
template< class U1, class U2 > tuple& operator=( const std::pair< U1, U2 >& p );//C++11 起, C++20 前
因此,std::tie可以用于pair的解包:
std::set< int > set;
std::set< int >::iterator iter;
bool result;
std::tie(iter, result) = set.insert(value);//解包 insert 的返回值為 iter 與 result
std::tie(std::ignore, result) = set.insert(value);//使用std::ignore忽略insert的返回pair中的第一個元素
注 :std::ignore 是令 std::tie 在解包 std::tuple 時作為不使用的參數的占位符使用,即忽略某些 tuple 中的某些返回值。
2. 批量賦值
std::tie 可以將多個變量的引用整合成一個 tuple,進而通過另外一個同類型的 tuple 進行批量賦值。
tuple< string, double, int > tup("idoit", 98.8, 1);
string name;
double score;
int rank;
//通過變量tup實現對name、score、rank的批量賦值操作
tie(name, score, rank) = tup;
3. 比較結構體
可以將結構體成員傳入std::tie,從而實現結構體的比較。
struct S {
int n;
std::string s;
float d;
bool operator< (const S& rhs) const
{
// 比較 n 與 rhs.n,
// 然后為 s 與 rhs.s,
// 然后為 d 與 rhs.d
return std::tie(n, s, d) < std::tie(rhs.n, rhs.s, rhs.d);
//注:由于tie返回的是一個 tuple,tuple的實現已經重載了operator< ,因此可以利用tuple的operator< ,進而實現結構體S的operator< 。
}
};
具體示例
#include < iostream >
#include < set >
#include < string >
#include < tuple >
using namespace std;
struct S {
int n;
string s;
float d;
bool operator< (const S& rhs) const {
// 比較 n 與 rhs.n,
// 然后為 s 與 rhs.s,
// 然后為 d 與 rhs.d
return tie(n, s, d) < tie(rhs.n, rhs.s, rhs.d);
}
};
int main() {
set< S > set_of_s;
S value1{42, "Test1", 3.14};
S value2{23, "Test2", 3.14};
set< S >::iterator iter;
bool result;
/************解包**********/
tie(iter, result) = set_of_s.insert(value1);
if (result) cout < < "Value1 was inserted successfullyn";
tie(std::ignore, result) = set_of_s.insert(
value2); // 使用std::ignore忽略insert的返回pair中的第一個元素
if (result) cout < < "Value2 was inserted successfullyn";
/***********結構體比較**********/
bool r = value1 < value2;
cout < < "value1 < value2 : " < < r < < endl;
/***********批量賦值**********/
tuple< string, double, int > tup("idoit", 98.8, 1);
string name;
double score;
int rank;
tie(name, score, rank) = tup;
cout < < name < < " " < < score < < " " < < rank < < endl;
return 0;
}
輸出結果:
Value1 was inserted successfully
Value2 was inserted successfully
value1 < value2 : 0
idoit 98.8 1
-
轉換器
+關注
關注
27文章
8728瀏覽量
147437 -
C++語言
+關注
關注
0文章
147瀏覽量
7008
發布評論請先 登錄
相關推薦
C++虛函數virtual詳解
c++中冒號(:)和雙冒號(::)的用法
如何在中斷C函數中調用C++
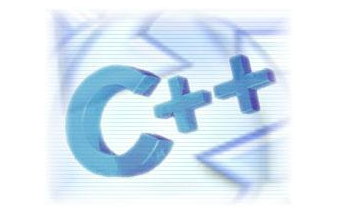
評論