鏈表是由一連串節點組成的數據結構,每個節點包含一個數據值和一個指向下一個節點的指針。鏈表可以在頭部和尾部插入和刪除節點,因此可以在任何地方插入和刪除節點,從而使其變得靈活和易于實現。
鏈表通常用于實現有序集合,例如隊列和雙向鏈表。鏈表的優點是可以快速隨機訪問節點,而缺點是插入和刪除操作相對慢一些,因為需要移動節點。此外,鏈表的長度通常受限于內存空間,因此當鏈表變得很長時,可能需要通過分頁或鏈表分段等方式來管理其內存。
下面是一套封裝好的單鏈表框架,包括創建鏈表、插入節點、刪除節點、修改節點、遍歷節點和清空鏈表等常見操作,其中每個節點存儲一個結構體變量,該結構體中包含一個名為data的int類型成員。
#include
#include
?
// 鏈表節點結構體
typedef struct ListNode {
int data; // 節點數據
struct ListNode *next; // 下一個節點的指針
} ListNode;
?
// 創建一個新節點
ListNode *createNode(int data) {
ListNode *node = (ListNode*) malloc(sizeof(ListNode));
node->data = data;
node->next = NULL;
return node;
}
?
// 在鏈表頭部插入一個新節點
ListNode *insertNodeAtHead(ListNode *head, int data) {
ListNode *node = createNode(data);
node->next = head;
return node;
}
?
// 在鏈表尾部插入一個新節點
ListNode *insertNodeAtTail(ListNode *head, int data) {
ListNode *node = createNode(data);
if(head == NULL) {
return node;
} else {
ListNode *current = head;
while(current->next != NULL) {
current = current->next;
}
current->next = node;
return head;
}
}
?
// 刪除鏈表中第一個值為data的節點
ListNode *deleteNode(ListNode *head, int data) {
if(head == NULL) {
return NULL;
}
if(head->data == data) {
ListNode *current = head;
head = head->next;
free(current);
return head;
}
ListNode *current = head;
while(current->next != NULL && current->next->data != data) {
current = current->next;
}
if(current->next != NULL) {
ListNode *deleteNode = current->next;
current->next = deleteNode->next;
free(deleteNode);
}
return head;
}
?
// 修改鏈表中第一個值為oldData的節點的數據為newData
void updateNode(ListNode *head, int oldData, int newData) {
ListNode *current = head;
while(current != NULL) {
if(current->data == oldData) {
current->data = newData;
break;
} else {
current = current->next;
}
}
}
?
// 遍歷鏈表
void traverseList(ListNode *head) {
ListNode *current = head;
while(current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("
");
}
?
// 清空鏈表,釋放所有節點的內存空間
void clearList(ListNode *head) {
while(head != NULL) {
ListNode *current = head;
head = head->next;
free(current);
}
}
?
// 示例程序
int main() {
ListNode *head = NULL;
head = insertNodeAtHead(head, 1);
head = insertNodeAtHead(head, 2);
head = insertNodeAtTail(head, 3);
traverseList(head);
head = deleteNode(head, 2);
traverseList(head);
updateNode(head, 1, 4);
traverseList(head);
clearList(head);
return 0;
}
在上述代碼中,定義了一個節點結構體ListNode,其中包含一個int類型的data成員和一個指向下一個節點的指針。接著定義了用于創建新節點、插入節點、刪除節點、修改節點、遍歷節點和清空鏈表等操作的子函數,并在main函數中演示了這些操作的使用例子。在使用完鏈表后一定要調用clearList函數釋放內存空間。
審核編輯:湯梓紅
-
內存
+關注
關注
8文章
3052瀏覽量
74309 -
C語言
+關注
關注
180文章
7614瀏覽量
137670 -
函數
+關注
關注
3文章
4345瀏覽量
62959 -
指針
+關注
關注
1文章
481瀏覽量
70603 -
數據結構
+關注
關注
3文章
573瀏覽量
40229
發布評論請先 登錄
相關推薦
Qt(C++)使用SQLite數據庫完成數據增刪改查
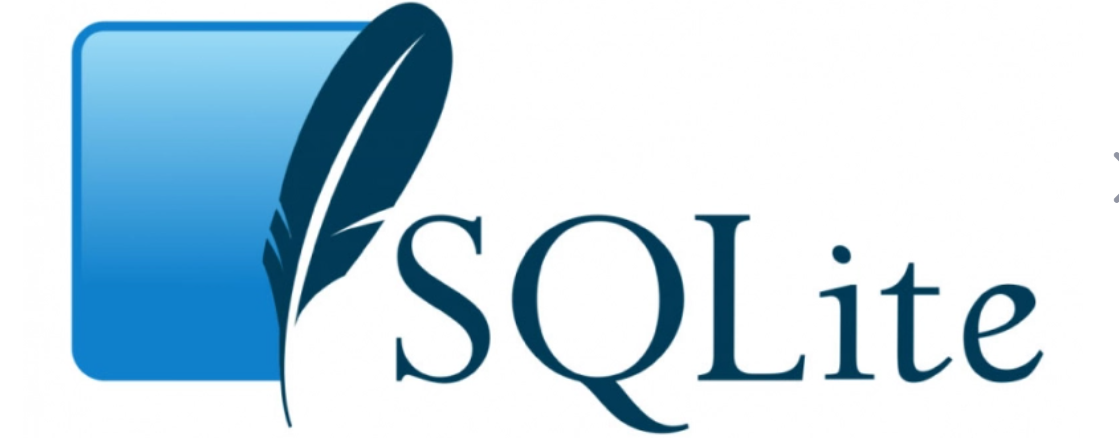
評論